
AT&T In-App Messaging API Documentation
Introduction
The In-App Messaging API sends, receives, updates, and deletes MMS and SMS messages on behalf of a specific user after confirming explicit user consent.
Using this API, your app can send MMS and SMS messages to another phone number (AT&T and cross-carrier), short codes, or e-mail addresses.
API Quick Start

Get App Key & App Secret
To start working with AT&T APIs, create a developer account or sign in to your existing account on the AT&T Developer Program website.
Click on the My Apps selection and set up a new app account by providing an Application Name. Then, choose one or both of the following from the list of available APIs: In-App Messaging-IMMN, In-App Messaging-MIM and click submit to get an App Key and App Secret.

Get OAuth Access Token
AT&T uses the OAuth 2.0 framework to obtain an OAuth access token as shown in this call flow. To obtain an access token, use the following OAuth sample code:
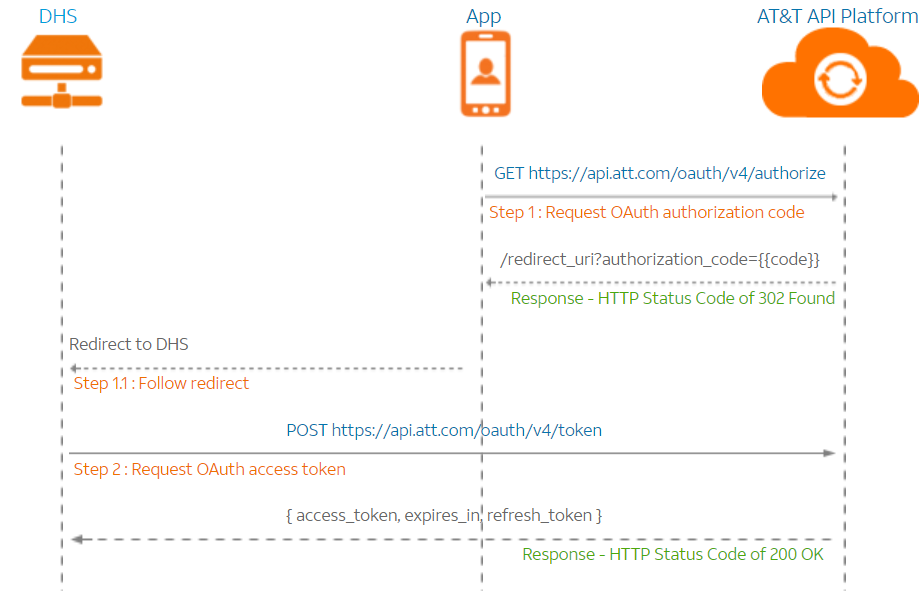
# Obtain these from your app account on the AT&T Developer Program website. APP_KEY="" APP_SECRET="" # Set up the scopes for requesting API access. SCOPE="IMMN,MIM" # Set up the redirect URL for consent flow. # Note that this must be escaped REDIRECT_URI="http%3A%2F%2Flocalhost" # Step 1: Request the OAuth authorization code from the user. echo "https://api.att.com/oauth/v4/authorize?client_id=${APP_KEY}&scope=${SCOPE}&redirect_uri=${REDIRECT_URI}" # Input the OAuth authorization code obtained from user consent. OAUTH_AUTHORIZATION_CODE="ENTER_VALUE" # Set up the payload. DATA="grant_type=authorization_code&client_id=${APP_KEY}&client_secret=${APP_SECRET}&code=${OAUTH_AUTHORIZATION_CODE}" # Step 2: Request the OAuth access token by exchanging the OAuth authorization code. curl "https://api.att.com/oauth/v4/token" \ --insecure \ --header "Content-Type: application/x-www-form-urlencoded" \ --header "Accept: application/json" \ --data ${DATA}
For more information refer to the OAuth 2.0 API.

Make API Calls
- Get Started - Send a Message
This scenario shows the most basic flow. Use it to send a message from the AT&T customer's message inbox to a phone number, short code or e-mail address. The message will appear as to be sent through the messaging app on the user's mobile device.
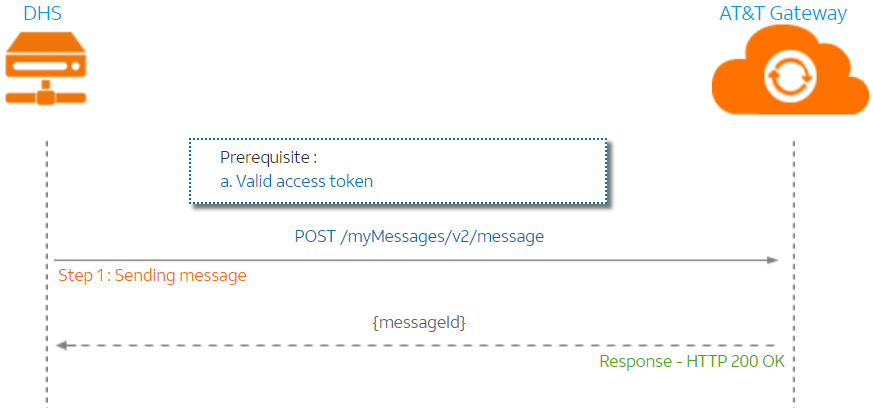
# Enter the authentication code generated by the consent flow here. OAUTH_ACCESS_TOKEN="ENTER_VALUE" # Telephone number to which the message is sent. TEL="ENTER_VALUE" # Message text body. MESSAGE="Message text" # Message text subject. SUBJECT="Message Subject" # Format the body that will be sent via curl. BODY={"Addresses":[${TEL}],"Text":"${MESSAGE}","Subject":"${SUBJECT}"} # Step 2: Send a message using the API. curl "https://api.att.com/myMessages/v2/messages" \ --header "Content-Type: application/json" \ --header "Authorization: Bearer ${OAUTH_ACCESS_TOKEN}" \ --header "Accept: application/json" \ --data "${BODY}" \ --request POST
- Read Inbox - Retrieve All Messages
This scenario shows how to retrieve all the messages from the user’s inbox. An index, on the message inbox, must exist before attempting to retrieve the messages. The index must be created once per inbox and may expire due to inactivity in the inbox. The offset parameter controls the starting position in the set of messages, and the limit parameter controls the number of messages retrieved.
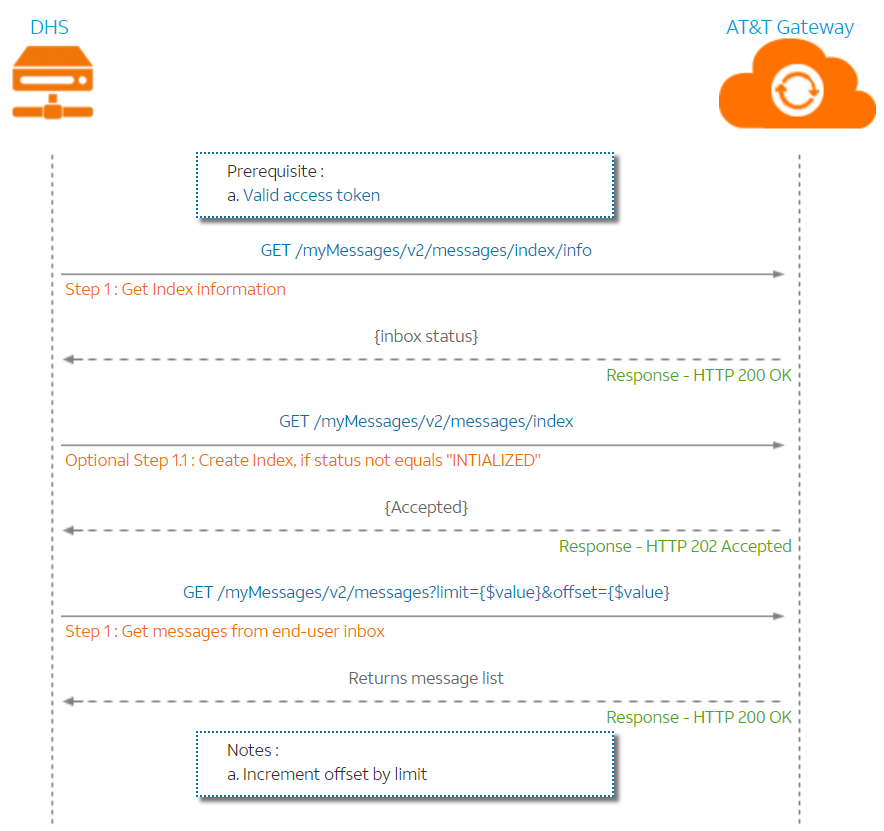
# Enter the authentication code generated by the consent flow here. OAUTH_ACCESS_TOKEN="ENTER_VALUE" # Enter a valid number (<= 300) of messages to request MESSAGE_LIMIT="100" # Step 2: Get a list of messages using the Get Message List method. curl "${FQDN}/myMessages/v2/messages?limit=${MESSAGE_LIMIT}" \ --header "Authorization: Bearer ${OAUTH_ACCESS_TOKEN}" \ --header "Accept: application/json" \ --request GET
OAuth
All APIs provided by AT&T use the OAuth 2.0 framework. All API requests sent to the API Gateway must include an OAuth access token.
You may authorize access for this API by using the following scopes.
- "IMMN" for the Send Message method.
- "MIM" for Message Inbox Management uses the Create Message Index method, the Delete Message method, the Delete Messages method, the Get Message method, the Get Message Content method, the Get Message Index Info method, the Get Message List method, the Get Messages Delta method, the Update Message method, and the Update Messages method.
For more information about the OAuth refresh token, other authentication options, and revoking tokens; see the OAuth 2.0 API v4.
Considerations
You need to be aware of the following considerations when using this API.
For any of the methods of this API, if an HTTP Status Code 503 is returned in the response, you should check the Retry-After header. If it exists, then the value given in the header indicates when it is recommended to retry the request.
For the Send Message method.
- This method sends messages only to mobile devices on other carriers in the USA. Sending messages to international numbers is not currently supported.
- There are a few limitations related to cache that are related to initialization and expiration. Please see our FAQs for more details.
For all of the methods.
- The consent from the user is required.
- The methods are only available to AT&T Wireless post-paid mobile phone users.
Managing a user's inbox.
- In order to utilize this API, the user must be provisioned with the AT&T; Cloud Inbox. This provisioning is done during the user consent authorization process. In the event that a user is not provisioned the first time, they can self-remedy the situation by trying to complete the user consent authorization for the app again at a later time. However, if the error persists, the customer should be advised to call AT&T CARE.
- The AT&T backup and sync service stores a maximum of 1000 SMS and MMS messages on the cloud for 90 days. Messages that are stored locally on devices are not deleted after that period
Managing a user's message index.
- In order to manage and update a user's message inbox, a message index must be created and maintained. Once it is created, the index is persisted for a 30-day rolling period, and can be kept alive beyond that time by sending a Get Message Index Info request once every 24 hours. There are practices that should be followed when managing the index in order to achieve higher performance and a better experience while interacting with the user's inbox.
Note: Due to platform enhancements in the November 2016 release, your index cache that was created previous to that release will either be new or will need to be re-created. In either case, the use of a previously stored state value will no longer work for delta calls. Your application will need to re-synchronize messages as required. Message IDs will be different. You may be able to correlate previously stored messages by using the timestamp and from/recipients to uniquely identify messages.
The developer must be aware that there are inactivity timers.
- Once the cache status is set to INITIALIZED, the cache status is persisted per customer for a 30-day rolling period when the Get Message Index Info method request is sent unless an error occurs in the AT&T Messaging system that corrupts the cache.
- A cache status that is set to INITIALIZED is continuously kept up-to-date with the source platform if the Get Message Index Info method request is sent during the last 24 hours. This guarantees that a long-running app will continue to receive notifications for deltas in the customer's AT&T Message inbox.
The main way to keep an app synchronized with the customer's AT&T Message inbox is described as following.
- A developer is also able to implement message polling using the Get Delta method request. This method sends only a summary of the updates to an AT&T Message inbox.
In the event that a customer's AT&T Message inbox is not able to be provisioned, in most cases, the customer is able self-remedy the situation by simply trying to complete the user consent authorization for the app again at a later time. However, if the error persists, the customer should be advised to call CARE to determine if the issue stems from a different issue or will take time to correct. The error message that the customer receives is similar to the following.
Sorry, we were unable to set up AT&T Messages Backup and Sync Service. Please try again later. If the error persists, please call Customer Care by dialing 611 from your AT&T mobile phone.
Create Message Index
The Create Message Index method creates an index cache for the user's AT&T Message inbox with prior user consent.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | POST https://api.att.com/myMessages/v2/messages/index |
Request
POST https://api.att.com/myMessages/v2/messages/index HTTP/1.1 host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 202 Accepted
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Delete Message
The Delete Message method deletes one specific message from a user's AT&T Message inbox with prior user consent.
Note: All In-App Messaging API methods that send, update or delete messages, operate asynchronously. For example, when sending a message, it may take a few seconds before the message is available to GET. Operations typically complete in less than one second.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | DELETE https://api.att.com/myMessages/v2/messages/{{messageId}} |
Request
DELETE https://api.att.com/myMessages/v2/messages/S335 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 204 No Content
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the specific message identifier that represents a message in the user's AT&T Message inbox to be deleted.
Delete Messages
The Delete Messages method deletes one or more messages from a user's AT&T Message inbox with prior user consent.
Note: All In-App Messaging API methods that send, update or delete messages, operate asynchronously. For example, when sending a message, it may take a few seconds before the message is available to GET. Operations typically complete in less than one second.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | DELETE https://api.att.com/myMessages/v2/messages |
Request
DELETE https://api.att.com/myMessages/v2/messages?messageIds=S335%2Ct456 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 204 No Content
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies one or more comma-delimited message identifiers that each represent a message in the user's AT&T Message inbox.
Note: If one or more of the specified messages are not deleted, then a response is returned that contains an error message with the list of the message identifiers that were not deleted.
Get Message
The Get Message method retrieves one specific message from the user's AT&T Message inbox.
For SMS messages, the text of the message is retrieved as well.
For MMS messages and content, use the Get Message Content method.
OAuth Scope | Scope: MMS | Model: authorization_code |
Resource | GET https://api.att.com/myMessages/v2/messages/{{messageId}} |
Request
GET https://api.att.com/myMessages/v2/messages/WI124 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
{ "message": { "messageId": "WU124", "from": { "value": "+12065551212" }, "recipients": [ { "value": "+14255551212" }, { "value": "someone@att.com" } ], "timeStamp": "2012-01-14T12:00:00", "isUnread": false, "type": "MMS", "typeMetaData": { "subject": "This is an MMS message with parts" }, "isIncoming": false, "mmsContent": [ { "contentType": "text/plain", "contentName": "part1.txt", "contentUrl": "https://api.att.com/myMessages/v2/messages/0", "type": "TEXT" }, { "contentType": "image/jpeg", "contentName": "sunset.jpg", "contentUrl": "https://api.att.com/myMessages/v2/messages/1", "type": "IMAGE" } ] } }
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies a message identifier that represents a message in the user's AT&T Message inbox.
An array of Message objects that contain the message details.
A Sender object that contains the sender for the message.
Indicates that the message is an incoming message and not an outgoing message.
Indicates that the message has not been read.
Specifies the unique identifier for the message.
An array of MMS Content objects that contain the multi-media message parts or attachments of the message.
Note: If the Type parameter is set to TEXT, then this parameter is not present or empty.
A Recipients object that contains the parties involved in the message.
Note: For outgoing messages, this parameter includes the phone numbers of all of the parties.
Note: For incoming messages, this parameter includes the phone numbers of all of the parties, except for the user's phone number.
Note: If the message is a group message, then this parameter may contain multiple addresses.
Specifies the date and time that the message was stored in the AT&T Message inbox.
Note: This parameter is specified using Coordinated Universal Time (UTC), formerly referenced as the Greenwich Mean Time (GMT) time zone.
Specifies the message type, either TEXT or MMS.
The acceptable values for this parameter are:
- TEXT : Used for SMS messages.
- MMS
A Type Meta Data object that contains the message-specific meta-data.
Specifies the sender involved in the message.
Specifies the content name for the specified message part or attachment.
Specifies the content type identifier for the message part or attachment.
Example: image/jpeg.
Specifies the resource URI path to retrieve the specified message part or attachment.
Specifies the multi-media type of the message part or attachment.
The acceptable values for this parameter are:
- AUDIO
- IMAGE
- TEXT
- VIDEO
Specifies the recipient involved in the message.
Indicates that the message is segmented into multiple parts, for SMS messages.
A Segmentation Details object that contains the details of the segmentation for the SMS message.
Specifies the subject for the message.
Note: This parameter is only applicable when the message type is MMS.
Specifies the reference number for messages that are part of the specified segmented SMS message.
Specifies the segmentation part number for the specified message.
Specifies the total number of segments for the specified segmented SMS message.
Get Message Content
The Get Message Content method retrieves media associated with a user message from the AT&T Message inbox using information returned by the Get Message List method request.
Note: As the messaging platform supports RCS communication, this operation can retrieve messages upto 10MB and in such cases the messages may use HTTP streaming and follow the HTTP Chunked encoding format.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | GET https://api.att.com/myMessages/v2/messages/{{messageId}}/parts/{{partId}} |
Request
GET https://api.att.com/myMessages/v2/messages/WI124/parts/1 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 200 OK content-type: image/jpeg content-length&: xxxx Date: Thu, 04 Jun 2009 02:51:59 GMT {{jpeg_binary_image_data}}
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the message identifier that represents a message in the user's AT&T Message inbox.
Specifies the content identifier that represents an attachment in the referenced user's message.
Get Message Index Info
The Get Message Index Info method retrieves the state, status, and message count of the index cache for the customer's AT&T Message inbox.
For MMS messages and content, use the Get Message Content method.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | GET https://api.att.com/myMessages/v2/messages/index/info |
Request
GET https://api.att.com/myMessages/v2/messages/index/info HTTP/1.1 host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 200 OK content-length: 711 content-type: application/json { "messageIndexInfo": { "status":"INITIALIZED", "state":"1388102635555", "messageCount":164 } }
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the decimal value, in octets, for both the entity-length and the transfer-length.
An octet is an 8-bit byte.
Specifies the representation format for the response body.
A Message Index Info object that contains the message index information for the user's AT&T Message inbox.
Specifies the number of message indexes cached for the user.
Specifies an opaque string that denotes the current state of the AT&T Message inbox.
Specifies the status of the message index cache in the orchestration.
The acceptable values for this parameter are:
- ERROR : An ERROR status requires the client to rebuild the cache.
- INITIALIZED
- INITIALIZING
- NOT_INITIALIZED
Get Message List
The Get Message List method retrieves meta-data for one or more messages from the customer's AT&T Message inbox.
The messages are sorted by timestamp, with the newest messages first.
For SMS messages, the text of the message is retrieved as well.
For MMS messages, use the Get Message Content method.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | GET https://api.att.com/myMessages/v2/messages |
Request
GET https://api.att.com/myMessages/v2/messages?limit=500&offset=50 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
{ "messageList": { "messages": [ { "messageId": "WU23435", "from": { "value": "+12065551212" }, "recipients": [ { "value": "+14255551212" }, { "value": "someone@att.com" } ], "timeStamp": "2012-01-14T12:00:00", "text": "This is a Group MMS text only message", "isUnread": false, "type": "TEXT", "typeMetaData": {}, "isIncoming": true, }, { "messageId": "WU123", "from": { "value": "+12065551212" }, "recipients": [ { "value": "+14255551212" } ], "timeStamp": "2012-01-14T12:01:00", "text": "This is an SMS message", "isUnread": false, "type": "TEXT", "typeMetaData": { "isSegmented": true, "segmentationDetails": { "segmentationMsgRefNumber": 112, "totalNumberOfParts": 4, "thisPartNumber": 1 } }, }, { "messageId": "WU124", "from": { "value": "+14255551212" }, "recipients": [ { "value": "+14255551212" }, { "value": "someone@att.com" } ], "timeStamp": "2012-01-14T12:00:00", "isUnread": false, "type": "MMS", "typeMetaData": { "subject": "Hello" }, "mmsContent": [ { "contentType": "text/plain", "contentName": "part1.txt", "contentUrl": "/myMessages/v2/messages/0", "type": "TEXT" }, { "contentType": "image/jpeg", "contentName": "sunset.jpg", "contentUrl": "/myMessages/v2/messages/1", "type": "MMS" } ] } ], "offset": 50, "limit": 10, "total": 3, "state": "1388102635555", "cacheStatus": "INITIALIZED", "failedMessages": [ "S334", "S443" ] } }
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Indicates that the returned set of messages are filtered based on whether each message is inbound or outbound.
Indicates that the returned set of messages are filtered based on whether each message is marked as unread or not.
Specifies the message receipient address as a filter criteria so that the messages that have receipients containing or matching the provided receipient address are returned.
Currently this parameter supports only a single phone number.
The number specified should be the sender in an incoming message or the recipient in an outgoing message.
Note: When the mobile number is passed as the search keyword, the prefix of +1 should not be included for North American numbers.
Specifies the upper limit of messages that would be returned in the response.
The acceptable values for this parameter are:
- 1 through 500
Specifies a comma-separated list of messageIds for the messages that must be retrieved from the AT&T Message inbox.
If this parameter is specified in the request, then this parameter value takes preference over any other parameters specified in the request and in fact the Messaging system ignores any other parameters specified in the requests.
If all of the message identifiers specified are not found, then a response containing an error message is returned.
If one or more, but not all, of the message identifiers specified are not found; then the message identifiers that are not found are listed in the failedMessages parameter in the a successful response.
Specifies the offset from the beginning of the ordered set of messages from where requested filters are applied.
A Message List object that contains the requested set of messages and message inbox-related meta-data.
Specifies the status of the message index cache.
The acceptable values for this parameter are:
- ERROR : An ERROR status requires the client to rebuild the cache.
- INITIALIZED
- INITIALIZING
- NOT_INITIALIZED
Specifies a list of one or more of the messages requested that do not exist in the AT&T Message Inbox, while the other messages that were requested do exist.
This parameter is returned only when the messageIds parameter is specified in the request for a specific array of messages.
Specifies the maximum number of messages requested for the response.
An array of Message objects that contain the messages and their related meta-data.
Specifies the offset from the beginning of the ordered set of messages.
Specifies the current state of the AT&T Message inbox.
Specifies the total number of messages to returned based on filter criteria provided in the request.
A Sender object that contains the sender for the message.
Indicates that the message is an incoming message and not an outgoing message.
Indicates that the message has not been read.
Specifies the unique identifier for the message.
An array of MMS Content objects that contain the multi-media message parts or attachments of the message.
Note: If the Type parameter is set to TEXT, then this parameter is not present or empty.
A Recipients object that contains the parties involved in the message.
Note: For outgoing messages, this parameter includes the phone numbers of all of the parties.
Note: For incoming messages, this parameter includes the phone numbers of all of the parties, except for the user's phone number.
Note: If the message is a group message, then this parameter may contain multiple addresses.
Specifies the date and time that the message was stored in the AT&T Message inbox.
Note: This parameter is specified using Coordinated Universal Time (UTC), formerly referenced as the Greenwich Mean Time (GMT) time zone.
Specifies the message type, either TEXT or MMS.
The acceptable values for this parameter are:
- TEXT : Used for SMS messages.
- MMS
A Type Meta Data object that contains the message-specific meta-data.
Specifies the sender involved in the message.
Specifies the content name for the specified message part or attachment.
Specifies the content type identifier for the message part or attachment.
Example: image/jpeg.
Specifies the resource URI path to retrieve the specified message part or attachment.
Specifies the multi-media type of the message part or attachment.
The acceptable values for this parameter are:
- AUDIO
- IMAGE
- TEXT
- VIDEO
Specifies the recipient involved in the message.
Indicates that the message is segmented into multiple parts, for SMS messages.
A Segmentation Details object that contains the details of the segmentation for the SMS message.
Specifies the subject for the message.
Note: This parameter is only applicable when the message type is MMS.
Specifies the reference number for messages that are part of the specified segmented SMS message.
Specifies the segmentation part number for the specified message.
Specifies the total number of segments for the specified segmented SMS message.
Get Messages Delta
The Get Messages Delta method provides the capability to check for updates by passing in a client state.
This is typically used when an app changes from being offline to becoming online.
If the user's AT&T Messaging inbox index cache does not exist, then an error message (such as MESSAGES.MESSAGES-4019) may be returned to the app and the app must re-initialize the cache.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | GET https://api.att.com/myMessages/v2/delta |
Request
GET https://api.att.com/myMessages/v2/delta?state=1388102635555 HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json
Response
HTTP/1.1 200 OK content-length: 711 content-type: application/json { "deltaResponse":{ "state":"1388102635555", "delta": [ { "adds": [ { "messageId": "t123", "isUnread": false }, { "messageId": "t456", "isUnread": false } ], "deletes": [ { "messageId": "t789", "isUnread": false } ], "type": "TEXT", "updates": [ { "messageId": "t222", "isUnread": false }, { "messageId": "t223", "isUnread": false } ] }, { "adds": [ ], "deletes": [ ], "type": "MMS", "updates": [ ] } ] } }
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the last known state identifier that uniquely represents the last state of the cache from which point the app must identify and recognize the delta changes.
The app should have this string from a previous response to the Get Message Index method request or the Get Message List method request.
Specifies the decimal value, in octets, for both the entity-length and the transfer-length.
An octet is an 8-bit byte.
Specifies the representation format for the response body.
An array of Delta Response objects that contain the response details with the new state identifier and the delta changes from the previous state of the AT&T Message inbox.
A Delta Changes object that contains the delta changes grouped by the action type (add, update, or delete) and message type (TEXT or MMS).
Specifies the current state of the message inbox.
An array of Message Details objects that contains the details about the new additions.
An array of Message Details objects that contain the details about the delta changes with respect to the deleted messages.
Specifies the type of the message for the listed change.
An array of Message Details objects that contain the details about the new updates.
Indicates that the message is marked as not read.
Specifies the identifier of the message that has been added, deleted, or updated.
Send Message
The Send Message method sends messages on behalf of an AT&T Wireless user.
Each time this method request is sent, a single message may be sent to one or more destination mobile devices.
Messages are processed synchronously and sent asynchronously to the destination mobile device. Note: All In-App Messaging API methods that send, update or delete messages, operate asynchronously. For example, when sending a message, it may take a few seconds before the message is available to GET. Operations typically complete in less than one second.
The request may have up to 21 attachments, but the total size of the message must be 1 megabyte (MB) or less.
There are two ways that an MMS message can be sent: by using a MIME-encoded message, or by embedding the Base64 encoded representation of the media in the JSON or XML element.
- This method sends messages as SMS or MMS as follows.
- For SMS Messages: Text only, using the ASCII character range.The acceptable maximum length is 160 characters.
- For MMS messages: Any attachment, or text with a length greater than 160 characters.
- This method supports the following MMS content types.
- application/vnd.wap.multipart.mixed - without SMIL component.
- application/vnd.wap.multipart.related - without SMIL component.
- application/vnd.wap.multipart.related - with SMIL component.
- This method is limited to the following content types for MMS messages.
- 3GPP
- AAC-LC
- AMR-NB
- ASCII
- GIF87a
- GIF89a
- JPEG
- MP3
- MP4
- PIM Support : vCalendar 1.0
- SP-MIDI
- UTF-8
- UTF-16
- V-card PIM
OAuth Scope | Scope: IMMN | Model: authorization_code |
Resource | POST https://api.att.com/myMessages/v2/messages |
- JSON
- JSON (embedded base64)
- JSON (media)
- URL Encoded
- URL Encoded (embedded base64)
- XML
- XML (embedded base64)
- XML (media)
Request
POST https://api.att.com/myMessages/v2/messages HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 content-type: application/json { "messageRequest": { "addresses": [ "tel:2085554321", "tel:2025556789", "short:234567", "developer@att.com" ], "text": "Hello world" } }
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the decimal value, in octets, for both the entity-length and the transfer-length.
An octet is an 8-bit byte.
Specifies the representation format for the request.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
A messageRequest object that contains the request message.
Specifies one or more destination addresses.
The acceptable format for this parameter is the protocol identifier (ID) followed by the URL-escaped AT&T mobile number, short code, or e-Mail address.
The country code and preceding plus ( + ) symbol are optional.
Note: The maximum number of supported e-mail addresses is 10.
Note: If any address is duplicated, then the request is sent only once to that address.
Example: tel%3A%2B16309700001
Example: tel%3A16309700001
Example: tel%3A6309700001
Example: short%3A309
Indicates the visibility of the recipients for message.
Note: If this parameter is set to true, then all recipients are visible and Reply-All is allowed.
Note: This parameter is only applicable if there are multiple recipients.
Note: If this parameter is not set, then the message is treated as Broadcast.
A Message Content object that contains the message when embedding media content within the JSON or XML payload.
Specifies the subject for the message.
Note: The maximum length for this parameter is 512 characters.
Specifies the text portion of the message being sent.
Note: If the Attachments parameter is not specified, then this parameter must be specified.
Specifies the logical reference to one or more attachments.
Note: Multiple attachments are supported as long as the size restrictions are not violated.
Note: This data becomes mandatory if the value in "Text" is not provided in the request.
Note: If the request is being sent with the media contents embedded in the JSON or XML document, then this references the base64-encoded string of the content.
Specifies the encoding of the payload for MMS messages.
Specifies the representation format for each content part in a MMS message.
Example: image/jpeg.
Specifies the string for the filename of the media content.
Note: This parameter is specified when embedding media content within the JSON or XML payload.
Specifies the unique identifier for the message that may be used for troubleshooting.
Update Message
The Update Message method updates some settings related to one of the messages in a user's AT&T Message inbox with prior user consent.
Note: All In-App Messaging API methods that send, update or delete messages, operate asynchronously. For example, when sending a message, it may take a few seconds before the message is available to GET. Operations typically complete in less than one second.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | PUT https://api.att.com/myMessages/v2/messages/{{messageId}} |
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the decimal value, in octets, for both the entity-length and the transfer-length.
An octet is an 8-bit byte.
Specifies the representation format of the request body.
The acceptable values for this parameter are:
- application/json
- application/xml
An Update Message Settings object that contains a user's message and the settings of a message in a customer's AT&T Message inbox.
Specifies the unique identifier for the message.
Indicates that the message is marked as not read.
Note: The request is not valid if this parameter is not present in the request.
Update Messages
The Update Messages method updates some settings related to one or more messages in a user's AT&T Message inbox with prior user consent.
Note: All In-App Messaging API methods that send, update or delete messages, operate asynchronously. For example, when sending a message, it may take a few seconds before the message is available to GET. Operations typically complete in less than one second.
OAuth Scope | Scope: MIM | Model: authorization_code |
Resource | PUT https://api.att.com/myMessages/v2/messages |
Request
PUT https://api.att.com/myMessages/v2/messages HTTP/1.1 Host: api.att.com authorization: Bearer xyz123456789 accept: application/json content-type: application/json Content-length: 99 { "messages": [ { "messageId":"a123", "isUnread":true }, { "messageId":"b456", "isUnread":true } ] }
Response
HTTP/1.1 204 No Content
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Specifies the decimal value, in octets, for both the entity-length and the transfer-length.
An octet is an 8-bit byte.
Specifies the representation format of the request body.
The acceptable values for this parameter are:
- application/json
- application/xml
A Messages object that contains one or more user's messages and related settings of the messages in a customer's AT&T Message inbox.
Specifies the unique identifier for the message.
An updateMessageSettings object that contains one or more user's messages and related settings of the messages in a customer's AT&T Message inbox.
Indicates that the message is marked as not read.
Note: The request is not valid if this parameter is not present in the request.
Sorry! Your session has expired.