
API Version

The SMS API requires free full access and special permission before it can be used. The API is intended for established businesses or organizations to send SMS Shortcode messages to AT&T subscribers within the United States.
Introduction
The SMS API sends SMS messages to one or more AT&T Wireless mobile phones in a single request.
This API contains methods for sending and receiving messages and querying for the status of previously submitted SMS messages. For Mobile Originating messages, the SMS API allows you to poll the delivery status of the message or request that delivery status notifications be sent to a registered callback listener URI as soon as the message arrives.
1. Get App Key & App Secret
To start working with AT&T APIs, create a developer account or sign in to your existing account on the AT&T Developer Program website.
Click on the My Apps selection to set up a new app account by providing an Application Name, choosing SMS from the list of available APIs and, clicking submit to get an App Key and App Secret.
Applicable Scopes : SMS
2. Get OAuth Access Token
AT&T uses the OAuth 2.0 framework to obtain an OAuth access token as shown in this call flow. To obtain an access token, use the following OAuth sample code:
cURL
# Obtain these from your account on the AT&T Developer Program website. APP_KEY="" APP_SECRET="" # Set up the scopes for requesting API access. API_SCOPES="SMS" curl "https://api.att.com/oauth/v4/token" \ --insecure \ --header "Accept: application/json" \ --header "Content-Type: application/x-www-form-urlencoded" \ --data "client_id=${APP_KEY}&client_secret=${APP_SECRET}&grant_type=client_credentials&scope=${API_SCOPES}"
For more information refer to the OAuth 2.0 API.
Send an SMS message from an app to a phone(s)
The Send SMS method sends an SMS message to one or more AT&T Wireless network mobile phone(s). A unique identifier (messageId) returned in the response may be used to query for the delivery status of the message.
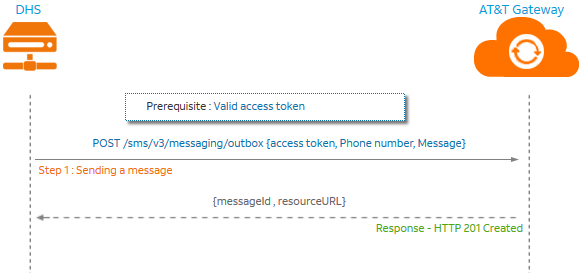
cURL
# Step 1: # Authentication parameter. For directions on how to obtain the OAuth access # token, see the OAuth section. OAUTH_ACCESS_TOKEN="ENTER_VALUE" # Step 2: # Fully qualified domain name for the API Gateway. FQDN="https://api.att.com" # SMS message text body. SMS_MSG_TEXT="SMS Message Text" # Enter telephone number to which the SMS message will be sent. # For example: TEL="tel:+1234567890" TEL="ENTER_VALUE" # Send the Send SMS method request to the API Gateway. curl "${FQDN}/sms/v3/messaging/outbox" \ --header "Content-Type: application/json" \ --header "Accept: application/json" \ --header "Authorization: Bearer ${OAUTH_ACCESS_TOKEN}" \ --data "{\"outboundSMSRequest\":{\"address\":\"${TEL}\",\"message\":\"${SMS_MSG_TEXT}\"}}" \ --request POST
Receive SMS messages via polling
The Get SMS method uses polling to periodically check for messages from the AT&T API Gateway.
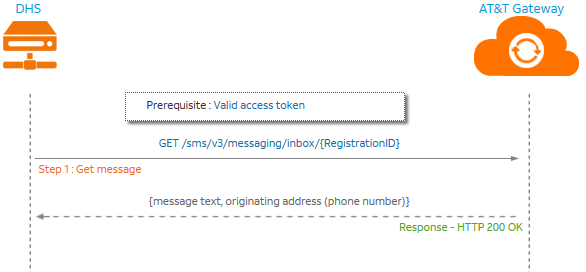
cURL
# Step 1: # Authentication parameter. For directions directions on how to obtain the OAuth access # token, see the OAuth section. OAUTH_ACCESS_TOKEN="ENTER_VALUE" # Step 2: # Fully qualified domain name for the API Gateway. FQDN="https://api.att.com" # Enter the registration identifier used to get an SMS message. REGISTRATION_ID="ENTER_VALUE" # Send the Get SMS method request to the API Gateway. curl "${FQDN}/sms/v3/messaging/inbox/${REGISTRATION_ID}" \ --header "Authorization: Bearer ${OAUTH_ACCESS_TOKEN}" \ --header "Accept: application/json" \ --request GET
Receive SMS messages via notification
The Receive SMS method receives notifications at the developer's listener URL, which is provisioned when an app is created. The Receive SMS callback enables an app to immediately receive mobile-originated SMS messages destined for the short codes assigned to the app.
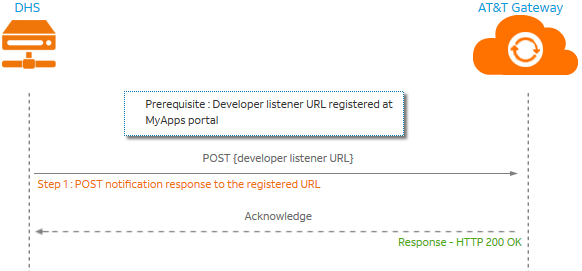
OAuth
All APIs provided by AT&T use the OAuth 2.0 framework. All API requests sent to the API Gateway must include an OAuth access token. You may authorize access for this API by using the following scope.
- "SMS" for the Get SMS method, the Get SMS Delivery Status method, the Receive SMS callback, the Receive SMS Delivery Status callback, and the Send SMS method.
To obtain an OAuth access token, use the App Key and App Secret from the My Apps section after registering and signing into your API Platform account. To obtain your OAuth access token run the following command from the Command Line Interface window and replace "{{APP_KEY}}", "{{APP_SECRET}}", and "{{API_SCOPES}}" with the App Key, App Secret, and the API scopes that are specified in your app account.
Get Access Token method using the cURL command:
curl "https://api.att.com/oauth/v4/token" \ --insecure \ --header "Accept: application/json" \ --header "Content-Type: application/x-www-form-urlencoded" \ --data "client_id={{APP_KEY}}&client_secret={{APP_SECRET}}&grant_type=client_credentials&scope={{API_SCOPES}}"
A successful response from the API Gateway contains an OAuth access token, the expiration period, and an OAuth refresh token as displayed in the following example.
Response from the API Gateway for the Get Access Token method:
HTTP/1.1 200 OK Content-Type: application/json Cache-Control: no-store Date: {{DATE_AND_TIME_STAMP}} { "access_token":"{{OAUTH_ACCESS_TOKEN}}", "token_type": "bearer", "expires_in":{{EXPIRATION_PERIOD}}, "refresh_token":"{{REFRESH_TOKEN}}" }
Extract the value from "{{OAUTH_ACCESS_TOKEN}}" to use in the Authorization parameter for requests to the API Gateway. When sending a request to the API Gateway a successfully constructed Authorization parameter looks like the following.
Authorization parameter for the HTTP header:
Authorization: Bearer {{OAUTH_ACCESS_TOKEN}}
For more information about the OAuth refresh token, other authentication options, and revoking tokens; see the OAuth 2.0 API v4.
Considerations
The RESTful SMS API provided by AT&T has the following considerations.
- You can send an SMS message to a maximum of 10 recipients at a time.
- All the messages sent are retried for up to 72 hours and any undelivered messages get deleted after that.
- AT&T supports two signatures of SMS API GSMA One and AT&T. If the application wants to use multiple short codes to send SMS messages, then consider using GSMA One API. Using the GSMA One API enables you to specify a short code as a parameter in the URI to indicate the originating sender address for the SMS message.
- Any SMS message that uses the Unicode encoding must be handled by the app by converting the hexadecimal value of the body using the first byte as the key for the encoding.
Provisioning
The RESTful SMS API provided by AT&T requires that you provision your app as follows.
- By default, an online short code is assigned to your application for the reception of Mobile Originating SMS messages via a callback URI, so that the application gets a notification as soon as a message is received. You must register this callback URI during provisioning.
- You can bring your own short code (custom short code) to use for your application. The process for on-boarding a custom short code typically takes 1-2 weeks. For more information, please see the FAQs.
- The GSMA One API can support multiple short codes for an application. When using the GMA One API to send an SMS message from the application, the sender address should always be set to the highest numbered short code available for the application.
- For delivery status of an SMS message you can have a callback or you can poll for the message.
AT&T
Overview
The Get SMS method receives multiple SMS messages sent to the short code associated with the app account from the AT&T Wireless network.
Each time this method is requested all SMS messages are received for the short code associated with the app account.
This method removes the messages from AT&T and therefore it retrieves only those SMS messages received since the previous Get SMS method request.
If there are more messages than could fit into a single response, the app must invoke multiple method requests to retrieve the complete list of the messages.
The totalNumberOfPendingMessages parameter in the SMS API response indicates that there are any more messages left on the server that are not sent in the current response.
An app that supports receiving SMS messages must invoke this request at least once every 30 minutes; otherwise the messages are automatically removed from the API Gateway.
For apps that expect to receive a lot of SMS messages, it is recommended that the app invokes this request frequently, but with a maximum frequency of one every 5 minutes.
Note: If the developer's app is expecting a huge number of Mobile Originated (MO) messages, then it is recommended to use the Receive SMS callback by registering a listener URI for the app where the API Gateway immediately delivers the incoming messages as soon as each message arrives in the AT&T Wireless network.
Note: This method is only allowed for a short code that is set up as an offline short code.
The process to obtain an offline short code for your app is described below.
Add one or more offline short codes as follows.
- Your first step depends upon your app account.
- If you have not created an SMS app account, then create an app account with the API set to SMS and click on the Manage Short Code link after submitting your app account.
- If you have already created an SMS app account, go to the My Apps page, click on the link to your SMS app account, and navigate to the Manage Short Code page.
- Click on the Add Short Code link and click the Save button without adding any URI information.
Note: If you want the SMS messages sent from the user's mobile device to be forwarded to your app immediately by the API Gateway, then you must register your SMS listener URI after creating the SMS app.
This enables you to utilize the Receive SMS method.
Note: This method removes all of the messages for the registrationID parameter value that are stored on the AT&T Wireless network at the time that this method is requested.
OAuth Scope
Scope: SMS
Model: client_credentials
Resource
GET https://api.att.com/sms/v3/messaging/inbox/{{RegistrationID}}
Request and Response Examples
JSON
Request
GET https://api.att.com/sms/v3/messaging/inbox/22627000 Authorization: Bearer xyz123456789 Accept: application/json
Response
{ "InboundSmsMessageList": { "InboundSmsMessage": [ { "MessageId": "msg0", "Message": "Hello", "SenderAddress": "tel:4257850159" } ], "NumberOfMessagesInThisBatch": "1", "ResourceUrl": "https://api.att.com/sms/v3/messaging/inbox/22627000", "TotalNumberOfPendingMessages": "0" } }
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
RegistrationID ★
String
URI Path
Specifies the identifier (ID) of the inbox which stores all the messages sent from an AT&T Wireless customer for a specified short code associated with the app.
Note: This ID always matches the short code for which the app is checking for any inbound messages.
GSMA OneAPI
Overview
The Get SMS method receives multiple SMS messages sent to the short code associated with the app account from the AT&T Wireless network.
Each time this method is requested all of the SMS messages are received for the short code associated with the app account.
This method removes the messages from AT&T and therefore it retrieves only those SMS messages received since the previous Get SMS method request.
If there are more messages than could fit into a single response, the app must invoke multiple method requests to retrieve the complete list of the messages.
The totalNumberOfPendingMessages parameter in the SMS API response indicates that there are any more messages left on the server that are not sent in the current response.
An app that supports receiving SMS messages must invoke this request at least once every 30 minutes; otherwise the messages are automatically removed from the API Gateway.
For apps that expect to receive a lot of SMS messages, it is recommended that the app invokes this request frequently, but with a maximum frequency of one every 5 minutes.
Note: If the developer's app is expecting a huge number of Mobile Originated (MO) messages, then it is recommended to use the Receive SMS callback by registering a listener URI for the app where the API Gateway immediately delivers the incoming messages as soon as each message arrives in the AT&T Wireless network.
Note: This method is only allowed for a short code that is set up as an offline short code.
The process to obtain an offline short code for your app is described below.
Add one or more offline short codes as follows.
- Your first step depends upon your app account.
- If you have not created an SMS app account, then create an app account with the API set to SMS and click on the Manage Short Code link after submitting your app account.
- If you have already created an SMS app account, go to the My Apps page, click on the link to your SMS app account, and navigate to the Manage Short Code page.
- Click on the Add Short Code link and click the Save button without adding any URI information.
Note: If you want the SMS messages sent from the user's mobile device to be forwarded to your app immediately by the API Gateway, then you must register your SMS listener URI after creating the SMS app.
This enables you to utilize the Receive SMS method.
Note: This method removes all of the messages for the registrationID parameter value that are stored on the AT&T Wireless network at the time that this method is requested.
OAuth Scope
Scope: SMS
Model: client_credentials
Resource
Request and Response Examples
XML
Request
GET https://api.att.com/3/smsmessaging/inbound/registrations/22627000/messages Authorization: Bearer xyz123456789 Accept: application/xml
Response
<inboundSMSMessageList> <inboundSMSMessage> <messageId>msg0</messageId> <message>Hello</message> <senderAddress>tel:+14257850159</senderAddress> </inboundSMSMessage> <numberOfMessagesInThisBatch>1</numberOfMessagesInThisBatch> <resourceURL>https://api.att.com/3/smsmessaging/inbound/registrations/22627012/messages</resourceURL> <totalNumberOfPendingMessages>0</totalNumberOfPendingMessages> </inboundSMSMessageList>
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
RegistrationID ★
String
URI Path
Specifies the identifier (ID) of the inbox which stores all the messages sent from an AT&T Wireless customer for a specified short code associated with the app.
Note: This ID always matches the short code for which the app is checking for any inbound messages.
Response Parameters
★Required Parameters
Parameter
InboundSmsMessageList ★
Object
Body
An Inbound SMS Message List object that contains the information for one or more SMS messages.
Inbound SMS Message List Object
★Required Parameters
InboundSmsMessage ★
Object
Body
An Inbound SMS Message object that contains the body of an inbound response.
Note: Multiple instances of this parameter may be present in the response.
NumberOfMessagesInThisBatch ★
String
Body
Specifies the number of messages sent in the batch response.
ResourceUrl ★
String
Body
Specifies the endpoint URI that serves this request.
TotalNumberOfPendingMessages ★
Integer
Body
Specifies the total number of messages pending that have not been retrieved from the server for the specific RegistrationId parameter value or short code.
Note: This parameter is based on a combination of size, the number of messages, and the maximum response size for the method request.
Note: The API Gateway may return messages in batches.
Note: If the messages are returned in batch mode due to a size limitation, then this parameter contains the remaining number of messages that are waiting to be retrieved from the server for the given short code.
Note: If this parameter value is greater than zero (0), then not all of the messages for a particular short code were returned in the response.
Note: In order to retrieve the messages that remain on the server, the app must make another request to retrieve the remaining messages.
Note: If this parameter value is zero (0) or empty, then there are no pending messages for the app to retrieve at this time.
Inbound SMS Message Object
★Required Parameters
Message ★
String
Body
Specifies the text of the message being sent.
Note: The maximum acceptable length for this parameter is 4096 1-byte characters.
MessageId ★
String
Body
Specifies the unique identifier for the message that is returned by the API Gateway.
SenderAddress ★
String
Body
Specifies the mobile device number of the sender or customer.
AT&T
Overview
The Get SMS Delivery Status method requests the status of a previously submitted SMS delivery request that was accepted by the API Gateway for delivery to the destination mobile device.
The Get SMS Delivery Status method is one of two ways in which the delivery status of a sent message may be obtained from the API Gateway.
The other way is to register an endpoint URI with the API Gateway which will be notified of the final status of the message. For a given message, only one of these mechanisms can be used.
The indicator of which mechanism will be used is the notifyDeliveryStatus parameter of the Send SMS method request.
To request the delivery status of a sent message using the Get SMS Delivery Status method, the notifyDeliveryStatus parameter of the Send SMS method must be set to false or should not be present in the request, this causes the messageId parameter value to be returned in the Send SMS method response.
The message identifier that was returned in the Send SMS method response must be provided in the Get SMS Delivery Status method request.
Note: The delivery status of the message is removed from the API Gateway and is not available for query, when one of the following events occurs.
- Thirty minutes have elapsed and the message has reached a final state of DeliveredToTerminal or DeliveryImpossible.
- The API Gateway responds to the first delivery status query after the message has reached the final state.
OAuth Scope
Scope: SMS
Model: client_credentials
Resource
GET https://api.att.com/sms/v3/messaging/outbox/{{messageId}
Request and Response Examples
JSON
Request
GET https://api.att.com/sms/v3/messaging/outbox/SMSa9b1927803784049 Authorization: Bearer xyz123456789 Accept: application/json
Response
{ "DeliveryInfoList": { "DeliveryInfo": [ { "Id": "msg0", "Address": "+13500000992", "DeliveryStatus": "DeliveredToTerminal" } ], "ResourceUrl": "https://api.att.com/sms/v3/messaging/outbox/SMSa9b192780378404c" } }
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
MessageId ★
String
URI Path
Specifies the unique identifier for the SMS message that is returned by the API Gateway.
GSMA OneAPI
Overview
The Get SMS Delivery Status method requests the status of a previously submitted SMS delivery request that was accepted by the API Gateway for delivery to the destination mobile device.
The message identifier returned in the response of the corresponding Send SMS method request must be provided in this method request.
Note: This method for polling for the delivery status message should work only when the notifyDeliveryStatus parameter is either set to false or not present in the Send SMS method request.
Note: The delivery status of the message is removed from the API Gateway and is not available for query, when one of the following events occurs.
- Thirty minutes have elapsed and the message has reached a final state of DeliveredToTerminal or DeliveryImpossible.
- The API Gateway responds to the first delivery status query after the message has reached the final state.
OAuth Scope
Scope: SMS
Model: client_credentials
Resource
Request and Response Examples
JSON
Request
GET https://api.att.com/3/smsmessaging/outbound/requests/80712002/SMSa9b1927803784049/deliveryInfos Authorization: Bearer xyz123456789 Accept: appication/json
Response
{ "deliveryInfoList": { "deliveryInfo": [ { "address": "tel:+13500000992", "deliveryStatus": "DeliveredToTerminal" } ], "resourceURL": "https://api.att.com/3/smsmessaging/outbound/requests/80712002/SMSa9b1927803784049/deliveryInfos" } }
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
requestId ★
String
URI Path
Specifies the unique identifier for the SMS message that is returned by the API Gateway.
SenderAddress ★
String
URI Path
Specifies the address that appears on the mobile device and to whom a responding message is sent.
Note: This parameter value should be the one of the short codes assigned to originating app account.
Note: This parameter is assignable only to the short code for the app that is sending the SMS message.
Response Parameters
★Required Parameters
Parameter
DeliveryInfoList ★
Object
Body
A Delivery Info List object that contains the body of the response.
Delivery Info List Object
★Required Parameters
DeliveryInfo ★
Object
Body
A Delivery Info object that contains the the delivery status of the SMS message.
ResourceUrl ★
String
Body
Specifies the URI being used to provide network delivery status of the sent message.
Note: This parameter is a self-referencing resource URI.
Delivery Info Object
★Required Parameters
address ★
String
Body
Specifies the destination address of the SMS message.
The acceptable format for this parameter is the term tel followed by a URL encoded colon character ( %3A ) followed by the URL encoded AT&T mobile number.
Note: The country code and plus sign character ( + ) are optional.
Example: "Address":"tel%3A%2B16309700001"
Example: "Address":"tel%3A16309700001"
Example: "Address":"tel%3A6309700001"
deliveryStatus ★
String
Body
Specifies the status of the SMS message that is delivered.
The acceptable values for this parameter are:
- DeliveredToNetwork : The message is delivered to the network, but not yet delivered to the user's mobile device.
- DeliveredToTerminal : The message is successfully delivered to the user's mobile device.
- DeliveryImpossible : The message is not successful delivered, such as when the message is not delivered before it expires.
Receive SMS
AT&T
Overview
The Receive SMS callback receives the Mobile Originated (MO) SMS messages in the app immediately for messages that are destined for the specified short codes of the app account.
In this case, the API Gateway acts as a client and the developer's app acts as a server.
The developer exposes the app functionality to the API Gateway in the form of a URI listener. This URI is used by the API Gateway to forward the received messages.
Note: The developer must register the URI or the system will not be able push the notification to the app for the Mobile Originated (MO) Messages destined for the short code.
Note: If the URI provided is HTTPS and port is not defined, then the default SSL port 443 will be used. The use of a transport mechanism other than HTTPS is not supported in the URI.
When a user sends a message to AT&T short code, the system determines the app to which the short code is associated, and then forwards the message to the listener URI provisioned with the short code of the app account.
The messages are removed from the system after delivery is acknowledged by the app or until all the retries as per the retry configuration are exhausted.
The app must respond to the notification with an appropriate HTTP Status Code class of 2xx for positive acknowledgment.
The following explanation for the handling of the HTTP Status Codes sent by the user's app is sent in response to a notification from the API Gateway.
- In case of the HTTP Status Code class of 2xx, the server assumes positive acknowledgement that the notification has been sent successfully.
- In case of no response, timeout condition or HTTP Status Code classes other than 2xx, the server assumes that either the API Gateway is temporarily unavailable or cannot receive and process the notification at that point in time.
If the app SMS Mobile Originated (MO) URI listener is unavailable, busy, or otherwise unable to process and acknowledge the Mobile Originated (MO) message, then it will be retried as per the retry configuration set in the network.
Typically the retry period is 72 hours after which the message will be discarded and no further retries would be attempted.
OAuth Scope
Scope: SMS
Model: Not Applicable
Resource
POST {{your_notification_callback_uri}
Notification Examples
JSON
Notification
{ "DateTime": "04-15-2011T12:00:00", "MessageId": "", "Message": "Hello world", "SenderAddress": "tel:+12071111111", "DestinationAddress": "52345678" }
Notification Parameters
★Required Parameters
Parameter
DateTime ★
String
Body
Specifies the time and date on which the message is received.
Example: "DateTime":"04-15-2011T12:00:00"
DestinationAddress ★
String
Body
Specifies the short code to which the message is destined.
Message ★
String
Body
Specifies the text of the message sent.
Note: The maximum acceptable length for this parameter is 4096 1-byte characters.
MessageId ★
String
Body
Specifies the unique identifier for the message that is returned by the API Gateway.
Note: For this release, this parameter is empty.
SenderAddress ★
String
Body
Specifies the mobile device number of the sender or customer.
The acceptable format for this parameter is the term tel followed by a URL encoded colon character ( %3A ) followed by a URL encoded plus sign character ( %2B ) followed by the URL encoded country code and AT&T mobile number
Example: "SenderAddress":"tel%3A%2B16309700001"
Receive SMS Delivery Status
AT&T
Overview
The Receive SMS Delivery Status callback receives notifications of the final delivery status of the SMS messages sent by the app.
In this case, the API Gateway acts as a client and the developer's app acts as a server.
The developer provides the app listener endpoint URI to the API Gateway during the app account registration process or with an update to the app account against the appropriate short code associated with the app.
There exists a one-to-one association between the URI and a short code.
The URI is used by the API Gateway to notify the final delivery status of the SMS messages that were earlier accepted for delivery by the API Gateway against a specified short code.
Note: The delivery notification is delivered to the app only if requested in the Send SMS method request by setting the notifyDeliveryStatus parameter value to true.
Note: There is only one notification message per destination address when the SMS message is being delivered to multiple recipients in a single request.
Note: All of the notifications in this case might not be necessarily at the same time as it depends on the delivery of the SMS message to each recipient.
Note: If the URI provided is HTTPS and port is not defined, then the default SSL port 443 is used.
The use of a transport mechanism other than HTTPS is not supported in the URI.
When an SMS message that was earlier accepted for delivery reaches the final state and the app has requested for delivery notification in the Send SMS method request, a notification message is sent to the endpoint URI registered by the app for the specified short code.
The SMS message reaches the final status when it is delivered to the user's mobile device or when it expires in the network after multiple retries.
The number of retries is based on the Network retry configuration, which is currently set to 72 hours.
If the notifyDeliveryStatus parameter value is set to true in the Send SMS method request and if the notification endpoint URI is not provided or associated to the corresponding short code of the app in the app account, then the delivery notification status message might become lost.
The app listener is expected to respond to the notification with the appropriate HTTP Status Code class of 2xx for positive acknowledgement.
The following explanations describe the handling of HTTP Status Codes sent by the user's app, in response to a notification from the server.
- In case of the HTTP Status Code class of 2xx, the server assumes positive acknowledgement that the notification has been sent successfully.
- In case of no response, timeout condition or HTTP Status Code classes other than 2xx, the server assumes that either the API Gateway is temporarily unavailable or cannot receive and process the notification at that point in time.
If the registered app delivery notification URI listener is unavailable, busy or otherwise unable to process and acknowledge the delivery status notification message then it will be retried as per the retry configuration set in the AT&T Wireless network.
Typically the retry period is 72 hours after which the message will be discarded and no further retries would be attempted.
OAuth Scope
Scope: SMS
Model: Not Applicable
Resource
POST {{your_notification_callback_uri}
Notification Examples
JSON
Notification
{ "deliveryInfoNotification": { "messageId": "0x181bce3883beadf85443215d6fL", "deliveryInfo": { "address": "tel:+13500000012", "deliveryStatus": "DeliveredToTerminal" } } }
Notification Parameters
★Required Parameters
Parameter
deliveryInfoNotification ★
Object
Body
A Delivery Info Notification object that contains the delivery status for a specific destination address.
Delivery Info Notification Object
★Required Parameters
DeliveryInfo ★
Object
Body
A Delivery Info object that contains the the delivery status of the SMS message.
MessageId ★
String
Body
Specifies the unique identifier for the message that is returned by the API Gateway.
Delivery Info Object
★Required Parameters
address ★
String
Body
Specifies the destination address of the SMS message.
The acceptable format for this parameter is the term tel followed by a URL encoded colon character ( %3A ) followed by the URL encoded AT&T mobile number.
Note: The country code and plus sign character ( + ) are optional.
Example: "Address":"tel%3A%2B16309700001"
Example: "Address":"tel%3A16309700001"
Example: "Address":"tel%3A6309700001"
deliveryStatus ★
String
Body
Specifies the status of the SMS message that is delivered.
The acceptable values for this parameter are:
- DeliveredToNetwork : The message is delivered to the network, but not yet delivered to the user's mobile device.
- DeliveredToTerminal : The message is successfully delivered to the user's mobile device.
- DeliveryImpossible : The message is not successful delivered, such as when the message is not delivered before it expires.
AT&T
Overview
The Send SMS method sends an SMS message to one or more AT&T Wireless mobile devices.
The SMS messages are processed synchronously and sent asynchronously to a destination on the AT&T Network.
A unique identifier (ID) is returned in the response that may be used to query the status of the message that has been sent.
Note: Using this provider of this method does not require you to specify the short code in your app account from which your SMS message will be originated.
The API Gateway uses the default short code associated with your app account as the originating sender address of the SMS message.
If you have more than one short code assigned to your app account and require the abilty to specify a specific short code as the originating sender address, then consider using the GSMA OneAPI provider of this method.
OAuth Scope
Scope: SMS
Model: client_credential
Resource
POST https://api.att.com/sms/v3/messaging/outbox
JSON (single recipient)
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/json { "outboundSMSRequest": { "address":"tel:+13500000992", "message":"Hello World", } }
Response
{ "outboundSMSResponse": { "messageId": "SMSa9b4dd85737c0409", "resourceReference": { "resourceURL": "https://api.att.com/sms/v3/messaging/outbox/SMSa9b4dd85737c0409" } } }
JSON (multiple recipient)
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/json { "outboundSMSRequest": { "address":[ "tel:+13500000992", "tel:+13500000993" ], "message":"Hello World", "notifyDeliveryStatus":true } }
Response
{ "outboundSMSResponse": { "messageId": "0x183269c8f460D1B3A170DF153FL" } }
JSON (notify delivery status)
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/json { "outboundSMSRequest": { "address":"tel:+13500000992", "message":"Hello World" “notifydeliverystatus”:True } }
Response
{ "outboundSMSResponse": { "messageId": "0x183269c8f460D1B3A170DF153FL", } }
URL Encoded
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/x-www-form-urlencoded address=tel%3A%2B13500000992%2Ctel%3A%2B13500000993& message=Hello%20World
URL Encoded (notify delivery status)
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/x-www-form-urlencoded address=tel%3A%2B13500000992%2Ctel%3A%2B13500000993& message=Hello%20World& notifyDeliveryStatus=True
XML
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/xml Content-Type: application/xml <outboundsmsrequest> <address>tel:+13500000992,tel:+13500000993</address> <message>HelloWorld</message> </outboundsmsrequest>
Response
<outboundsmsresponse messageid="SMSc04091ed284f5684"> <resourcereference> <resourceurl>https://api.att.com/sms/v3/messaging/outbox/SMSa9b4dd85737c0409</resourceurl> </resourcereference> </outboundsmsresponse>
XML (notify delivery status)
Request
POST https://api.att.com/sms/v3/messaging/outbox HTTP/1.1 Host: api.att.com Authorization: Bearer xyz123456789 Accept: application/xml Content-Type: application/xml <outboundSMSRequest> <address>tel:+13500000992,tel:+13500000993</address> <message>HelloWorld</message> <notifyDeliveryStatus>True</notifyDeliveryStatus> </outboundSMSRequest>
Response
<outboundSMSResponse messageId="0x183269c8f460D1B3A170DF153FL" />
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request body.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
outboundSMSRequest ★
Object
Body
An Outbound SMS Request object that contains the SMS message request.
GSMA OneAPI
Overview
The Send SMS method sends an SMS message to one or more AT&T Wireless mobile devices.
The SMS messages are processed synchronously and sent asynchronously to a destination on the AT&T Network.
A unique identifier (ID) is returned in the response that may be used to query the status of the message that has been sent.
Note: Using this provider of this method enables you to specify the sender originator address of the SMS message as a parameter in the URI, if you have one or more short codes assigned to your app account.
OAuth Scope
Scope: SMS
Model: client_credential
Resource
POST https://api.att.com/3/smsmessaging/outbound/{{senderAddress}}/requests
Request and Response Examples
JSON
Request
POST https://api.att.com/3/smsmessaging/outbound/80071200/requests Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/json { "outboundSMSRequest": { "address":[ "tel:+13500000992" ], "message":"GSMA OneAPI signature" } }
Response
{ "outboundSMSResponse": { "messageId": "SMSa9b690122ef1d729", "resourceReference": { "resourceURL": "https://api.att.com/3/smsmessaging/outbound/requests/80712002/SMSa9b690122ef1d729/deliveryInfos" } } }
JSON (notify delivery status)
Request
POST https://api.att.com/3/smsmessaging/outbound/80071200/requests Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/json { "outboundSMSRequest": { "address":[ "tel:+13500000992", "tel:+13500000993" ], "message":"GSMA OneAPI signature", "notifyDeliveryStatus":true } }
Response
{ "outboundSMSResponse": { "messageId": "0x183269c8f460D1B3A170DF153FL" } }
URL Encoded
Request
POST https://api.att.com/3/smsmessaging/outbound/80071200/requests Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/x-www-form-urlencoded address=tel%3A%2B13500000992%2Ctel%3A%2B13500000993& message=Hello%20World
Response
<outboundSMSResponse messageId="SMSa9b690122ef1d729"> <resourceReference> <resourceURL>https://api.att.com/3/smsmessaging/outbound/requests/80712002/SMSa9b690122ef1d729/deliveryInfos</resourceURL> </resourceReference> </outboundSMSResponse>
URL Encoded (notify delivery status)
Request
POST https://api.att.com/3/smsmessaging/outbound/80071200/requests Authorization: Bearer xyz123456789 Accept: application/json Content-Type: application/x-www-form-urlencoded address=tel%3A%2B13500000992%2Ctel%3A%2B13500000993& message=Hello%20World& notifyDeliveryStatus=True
Response
<outboundSMSResponse messageId="0x183269c8f460D1B3A170DF153FL"/>
Request Parameters
★Required Parameters
Parameter
Accept
String
Header
Specifies the format of the body for the response.
The acceptable values for this parameter are:
- application/json
- application/xml
The default value is application/json.
Note: For this method, this parameter specifies how the entity should be represented in case of an error.
This parameter is for setting the format of an error message. If there is no error, then the representation matches the form of the actual content.
Authorization ★
String
Header
Specifies the authorization.
The acceptable format for this parameter is the word "Bearer" followed by a space ( ) and an OAuth access token.
If this parameter value is missing from the header, then the API Gateway returns a message with an HTTP Status Code of 400 Invalid Request.
If the OAuth access token is not valid, then the API Gateway returns an HTTP Status Code of 401 Unauthorized with a WWW-Authenticate HTTP header.
Content-Type ★
String
Header
Specifies the representation format of the request body.
The acceptable values for this parameter are:
- application/json
- application/xml
- application/x-www-form-urlencoded
outboundSMSRequest ★
Object
Body
An Outbound SMS Request object that contains the SMS message request.
SenderAddress ★
String
URI Path
Specifies the address that appears on the user's mobile device and to whom a responding message is sent.
Note: This parameter value must be the one of the short codes assigned to your app account.
Note: This parameter is assignable only to the short code for the app that is sending the SMS message.
Note: App messaging is only supported using a short code.
Outbound SMS Request Object
★Required Parameters
address ★
Array of Strings
Body
Specifies one or more destination addresses of the message.
The acceptable format for this parameter is the term tel followed by a URL encoded colon character( %3A ) followed by the URL encoded AT&T mobile number.
Note: The country code and URL encoded preceding plus sign character ( %2B ) are optional.
Example: "Address":"tel%3A%2B16309700001"
Example: "Address":"tel%3A16309700001"
Example: "Address":"tel%3A6309700001"
Message ★
String
Body
Specifies the text of the message being sent.
Note: The maximum acceptable length for this parameter is 4096 1-byte characters.
notifyDeliveryStatus
Boolean
Body
Indicates that a delivery status notification is expected by the app.
If this parameter is set to "True", then the AT&T API Gateway sends a push notification to indicate the final delivery status of this message. The notification is sent to the endpoint URI registered against the given short code in your app’s short code management profile. If there is more than one recipient specified in the address parameter, one notification is sent per recipient.
If this parameter value is set to "False", then your app does not receive any delivery status notifications from the AT&T API Gateway.
Note
Note:If this flag is set to "True" but you have not registered any endpoint URI, a bad request error is thrown back in the message response.
Note
: This parameter is not part of the GSMA One API specification for this method. This is an additional parameter that is added to the AT&T version of the GSMA One API signature.
Response Parameters
★Required Parameters
Parameter
outboundSMSRequest ★
Object
Body
An Outbound SMS Response object that contains the body of the response.
Outbound SMS Response Object
★Required Parameters
MessageId ★
String
Body
Specifies the unique identifier for the message.
Note: This parameter value has two different purposes based upon the scenario.
- If the notifyDeliveryStatus parameter is either set to false or not present in the request, then this parameter value is used as a parameter in the URI on the Get SMS Delivery Status method to obtain the delivery status of the message.
- If the notifyDeliveryStatus parameter is set to true in the request, then this parameter value is returned in the delivery status callback notification sent to the registered listener URI for the app.
resourceReference ★
Resource Reference Object
Body
A Resource Reference object that contains the location of the delivery information.
Resource Reference Object
★Required Parameters
ResourceUrl ★
String
Body
Specifies the URI that provides network delivery status of the sent message.
Sorry! Your session has expired.